Tutorial : My first plugin¶
Note
The full code tutorial is provided here.
Setting up the environment¶
Create a new Pydev Project named my.company.first_plugin.
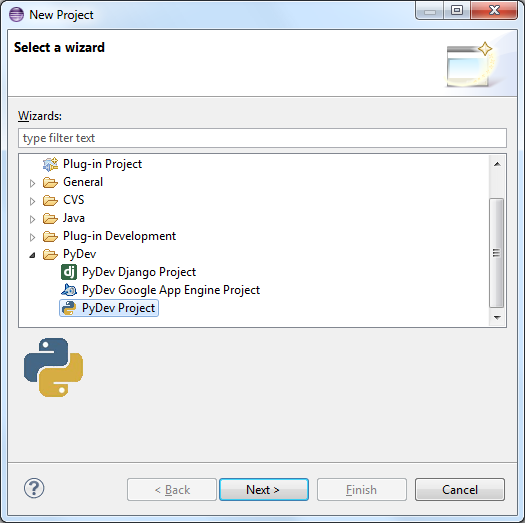
Create a project for your plugin.
Choose 3.0 for Grammar Version and the interpreter you declared before.
Create the plugin¶
In that project create a python module named main.
Create your plugin class in main.py:
import cast.analysers.dotnet
import cast.analysers.log
class MyExtension(cast.analysers.dotnet.Extension):
def start_analysis(self,options):
cast.analysers.log.debug('Hello World!!')
This will automatically register a .Net extension and create a plugin.
Create your first test¶
You can then test your plugin directly from eclipse.
In your project create a pydev package named tests. In that new package create a new module named basic_test and choose Module: Unittest as template.
Give the test method the name you like, we will start by launching a DotNet analysis in a test environment:
import unittest
import cast.analysers.test
class Test(unittest.TestCase):
def testRegisterPlugin(self):
# create a DotNet analysis
analysis = cast.analysers.test.DotNetTestAnalysis()
# DotNet need a selection of a csproj
analysis.add_selection('AProject/AProject.csproj')
analysis.set_verbose()
analysis.run()
if __name__ == "__main__":
unittest.main()
Select basic_test.py and with right click do Run As > Python Run
The test should then run and print the CAIP analysis log to console. Among the other analysis messages you should see:
...
Registering plugin my.company.first_plugin.
...
[my.company.first_plugin] Hello World!!
It means that it works.
Note
What we did here is to analyse a sample DotNet csproj. We have stored this sample directly inside the tests folder.
The analysis is performed whithout cast-ms. There is no need to have any CAIP databases installed.
The idea is that developper can tune it’s plugin code rapidly and build a full non regression test suite.
Now you can override other events.
Perform some checks on analysis results¶
When using the developement environment, analysis results are stored into temporary files and are accessible from inside unit tests.
After the run of the analysis we cna check for presence or absence of objects and links.
For example:
def testCheckInheritanceLink(self):
# create a DotNet analysis
analysis = cast.analysers.test.DotNetTestAnalysis()
# DotNet need a selection of a csproj
analysis.add_selection('AProject/AProject.csproj')
analysis.set_verbose()
analysis.run()
# perform some checks
my_class = analysis.get_object_by_name('AClass','CAST_DotNet_ClassCSharp')
self.assertTrue(my_class)
object_class = analysis.get_object_by_fullname('System.Object', 'CAST_DotNet_ClassExternal')
self.assertTrue(object_class)
link = analysis.get_link_by_caller_callee('inheritExtendLink', my_class, object_class)
self.assertTrue(link)
In that sample we check for the presence of two classes and of a link between them.
In debugging mode, the whole result is accessible through analysis.result.